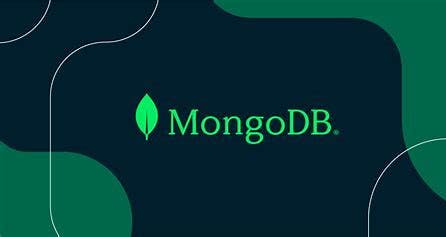
Transactions in MongoDB 101
March 27, 2024
Transactions in MongoDB are operations that allow you to execute multiple read and write operations across multiple documents in multiple collections as a single atomic operation.
Latest in tech
Software Dev
MongoDB
Introduction
Transactions in MongoDB are operations that allow you to execute multiple read-and-write operations across multiple documents in multiple collections as a single atomic operation.
Atomic means the transaction is treated as a single unit of work; either all of its operations are executed, or none are.
Why Use Transactions?
In many applications, it's important to ensure data integrity. Let's say you're building a banking application. When transferring money from one account to another, you need to decrease the balance in one account and increase it in another. It's crucial that either both of these operations succeed or neither does. If only one operation succeeds due to an error or system crash, the data would become inconsistent. Transactions help prevent such issues by ensuring atomicity.
How Do Transactions Work in MongoDB?
Transactions in MongoDB work similarly to transactions in traditional relational databases like MySQL, PostgresSql, etc. They follow the ACID properties:
- Atomicity : Ensures that all operations within a transaction are completed successfully. If any operation fails, the transaction is aborted, and no changes are made to the database.
- Consistency : Ensures the database remains in a consistent state before and after the transaction. Any changes made to a database must adhere to the database’s existing constraints, otherwise the whole transaction will fail. If, for example, one of the operations violated a schema validation rule, MongoDB would abort the transaction. This is to avoid multiple changes.
- Isolation : Ensures that multiple transactions occurring at the same time do not impact each other's execution. This means that if two transactions are executed simultaneously, the isolation rule guarantees the end result will be the same as if they were executed one after another.
- Durability : Ensures that once a transaction is committed, the changes are permanent, even in the case of a system failure.
A Real world Use Case Of Transactions in MongoDB
Let's consider a simple example using a banking application where you need to transfer $10 from Debby's account to Cynthia's account. Here are the steps I'll take:
- Start a Transaction : Begin the transaction to group the following operations.
- Check if Debby is rich enough : If she has enough balance ($10) to transfer.
- Deduct The money ($10) from Debby
- Credit Cynthia
- Commit the Transaction : If all operations are successful, commit the transaction to make the changes permanent.
- Abort if anything fails : If any operation within the transaction fails, abort the transaction, reverting any changes made since the transaction began.
Code Snippet For The Example Above
Note : The Code below is written in Node.js because, that's what I know. You can convert to your favourite language using ChatGPT or learn Node.js 🙃
const { MongoClient } = require('mongodb');
async function transferFunds(fromAccountId, toAccountId, amount) {
const client = new MongoClient('mongodb://localhost:27017');
try {
await client.connect();
const database = client.db('banking');
const accounts = database.collection('accounts');
const session = client.startSession();
// Start a transaction
session.startTransaction();
// Deduct amount from sender's account
await accounts.updateOne({ _id: fromAccountId }, { $inc: { balance: -amount } }, { session });
// Add amount to recipient's account
await accounts.updateOne({ _id: toAccountId }, { $inc: { balance: amount } }, { session });
// Attempt to commit the transaction
await session.commitTransaction();
console.log("Transfer successful!");
} catch (error) {
console.error("Transfer failed:", error);
// Abort the transaction in case of error
await session.abortTransaction();
} finally {
// End the session
await session.endSession();
// Close the connection
await client.close();
}
}
// Example usage
transferFunds('AliceAccountId', 'BobAccountId', 100);
In this example, if either the deduction or addition fails, the transaction is aborted, and the accounts are left unchanged, ensuring the atomicity of the operation.
Conclusion
MongoDB's transactions are powerful features that can help maintain data integrity in applications requiring multiple related operations to succeed or fail as a unit. They are particularly useful in applications dealing with financial data, order management systems, and else where data consistency is crucial. To learn more , you can checkout the Mongodb Docs. If you have corrections to make to this article, please feel free to express them in the comment.
See moreLeave a Reply
Your email address will not be published.
Required fields are marked*
Comment *
Name*
Email*