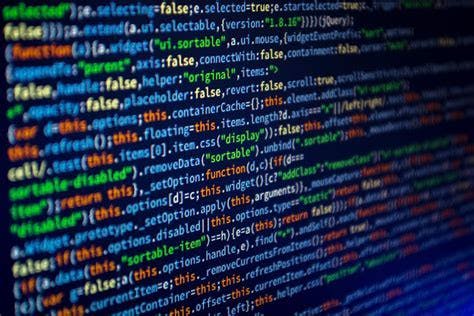
What are Stack and Heap in JavaScript?
In JavaScript, stack and heap are two memory spaces used for different purposes during program execution...
In JavaScript, Stack and Heap are two memory spaces used for different purposes during program execution.
Stack:
The stack is a special region of memory where variables are stored in a LIFO (Last-In-First-Out) order. It's a sequential data structure with two main operations: push (adds an item to the top of the stack) and pop (removes an item from the top of the stack).
- Execution Context: Each time a function is called, a new execution context is created and pushed onto the call stack. This context contains information such as local variables, parameters, and the return address.
- Function Calls: As functions are called within other functions, each function call adds a new frame to the call stack.
- Managing Function Calls: When a function finishes executing, its execution context is popped off the stack, and control returns to the calling function.
Heap:
The heap is a larger, more free-form region of memory used for dynamic memory allocation. It's a pool of memory where objects, arrays, and other data structures are stored. Unlike the stack, which has a fixed size and a strict order of operations, the heap is more flexible.
- Object Storage: Objects and complex data structures are typically allocated on the heap. When you create an object using new keyword or literals, the memory for that object is allocated on the heap.
- Dynamic Memory Allocation: Memory allocation and deallocation on the heap are more flexible compared to the stack. Objects can be created and destroyed dynamically, and memory can be allocated as needed.
Why Understanding Stack and Heap Matters?
Understanding the stack and heap is crucial for writing efficient and memory-safe JavaScript code.
- Memory Management: Knowing how memory is managed helps optimize memory usage and avoid memory leaks.
- Scope and Lifetime: Understanding the stack helps understand variable scope and lifetime, especially when dealing with closures and recursion.
- Performance: Efficient use of stack and heap memory can improve the performance of your JavaScript applications.
Example:
In this example, the variable x is stored on the stack, while the array arr and object obj are stored on the heap.
function foo() {
let x = 10; // variable x is stored on the stack
let arr = [1, 2, 3]; // array arr is stored on the heap
let obj = {a: 1, b: 2}; // object obj is stored on the heap
}
foo();
Conclusion:
Understanding the stack and heap in JavaScript helps developers write more efficient, memory-safe code. By knowing how variables are stored and managed, you can optimize memory usage and improve the performance of your applications.